Here is the Arduino BBB connected with the Adafruit Ethernet shield:
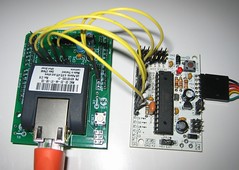
One important issue if you get the Adafruit Ethernet shield, it will be much more useful if you have an Arduino with an ATmega168, since then it can use the
AF SoftSerial library.
You see, the ATmega only has one hardware serial port, and you are generally busy using that to upload programs and monitor Arduino operation during testing. Lady Ada has put together a software serial library that doesn't suck (unlike the standard Arduino software serial library), but it depends on having an ATmega168 rather than an ATmega8.
If you have an old Arduino (like the NG), you can pick up a
pre-programmed ATmega168 from Adafruit to swap for your old ATmega8.
Here is my simplified version of a Lady Ada Xport example, creating a general "httpget" example. And yes, it works!
#include "AFSoftSerial.h"
#include "AF_XPort.h"
#include #include
char linebuffer[256];
int lines = 0;
#define XPORT_RXPIN 2
#define XPORT_TXPIN 3
#define XPORT_RESETPIN 4
#define XPORT_DTRPIN 0 // I'm not using DTR
#define XPORT_CTSPIN 6
#define XPORT_RTSPIN 7
AF_XPort xport = AF_XPort(XPORT_RXPIN, XPORT_TXPIN, XPORT_RESETPIN, XPORT_DTRPIN, XPORT_RTSPIN, XPORT_CTSPIN);
uint8_t errno,ret;
uint32_t laststatus = 0, currstatus = 0;
void setup() {
Serial.begin(57600);
xport.begin(9600);
}
void loop()
{
ret = httpget("64.233.183.104",80,"www.google.com","/");
if (ret)
Serial.println("get successful");
else
Serial.println("not successful");
delay(10000);
}
uint8_t httpget(char *ipaddr,
int port,
char *hostname,
char *httppath) {
uint8_t ret;
uint8_t success = 0;
ret = xport.reset();
Serial.print("Ret: "); Serial.print(ret, HEX);
switch (ret) {
case ERROR_TIMEDOUT: {
Serial.println("Timed out on reset!");
return 0;
}
case ERROR_BADRESP: {
Serial.println("Bad response on reset!");
return 0;
}
case ERROR_NONE: {
Serial.println("Reset OK!");
break;
}
default:
Serial.println("Unknown error");
return 0;
}
// time to connect...
ret = xport.connect(ipaddr, port);
switch (ret) {
case ERROR_TIMEDOUT: {
Serial.println("Timed out on connect");
return 0;
}
case ERROR_BADRESP: {
Serial.println("Failed to connect");
return 0;
}
case ERROR_NONE: {
Serial.println("Connected..."); break;
}
default:
Serial.println("Unknown error");
return 0;
}
xport.print("GET ");
xport.print(httppath);
xport.println(" HTTP/1.1");
xport.print("Host: "); xport.println(hostname);
xport.println("");
success=0;
while (1) {
// read one line from the xport at a time
ret = xport.readline_timeout(linebuffer, 255, 3000); // 3s timeout
// if we're using flow control, we can actually dump the line at the same time!
Serial.println(linebuffer);
if (strstr(linebuffer, "HTTP/1.1 200 OK") == linebuffer)
success = 1;
if (errno == ERROR_TIMEDOUT)
Serial.println("ERROR_TIMEDOUT");
if (xport.disconnected())
Serial.println("xport.disconnected");
if (((errno == ERROR_TIMEDOUT) && xport.disconnected()) ||
((XPORT_DTRPIN == 0) &&
(linebuffer[0] == 'D') && (linebuffer[1] == 0))) {
Serial.println("\nDisconnected...");
return success;
}
}
}
1 comment:
You code doesnt work
Post a Comment